Integrating SAWO Labs Authentication + Create React App
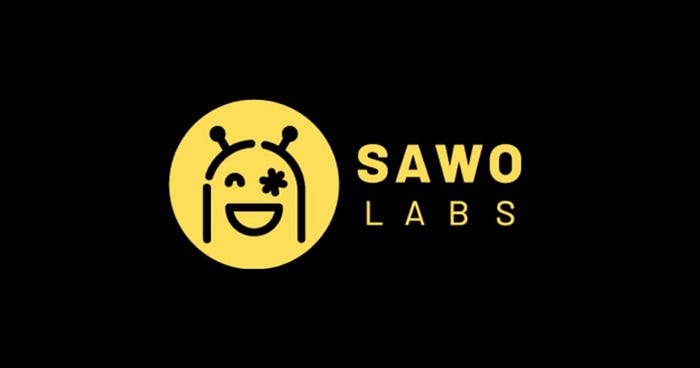
Hello there! I was working on building a simple authentication application using SAWO Labs. During the process, I found that there was a need to know more than what the official documentation provided.
Here then is a brief walkthrough to help you integrate SAWO Labs authentication with a React application.
The video version of the live code will be coming up on my Youtube
In Our small app:
- We are going to have a login page.
- We are going to have a dashboard page.
- Only an authenticated user can enter the dashboard page.
- We are going to use VSCode, and NPM as our package manager.
Let’s start
- You need a SAWO Labs account in order to use this awesome stuff. Head over to sawolabs.com and create your first project, and set the Host to
localhost
, if you chose to develop locally. Otherwise, you can set Host as your application URL. - You may use CodeSandbox React template, or if you are doing it locally, use the following command:
npx create-react-app client
. - 10 minutess later…
- We need to have the SAWO labs package in our
node_modules
. So head over to the terminal, make sure, you have enteredcd client
and run the following command to install the SAWO package:npm i sawo
. - Once the package is installed, you shall head over to
src/app.js
. - Clean the file by removing unnecessary code and the final look of src/app.js should look like this:
/* src/app.js */import React from "react";
import "./App.css";
function App() {
return (
<div id="App"> </div>
);
}export default App;
- Let’s import the SAWO package.
import React from "react";
import "./App.css";
import Sawo from "sawo";
- Save your API Key in the
.env.local
file and make sure you have added it before itself in.gitignore
. The.env.local
file should look like this:
REACT_APP_API_SAWO=11example1-821fexampled23-19830-318example2312
- Let’s head over to
src/app.js
. Based on the 5th point in the docs here. Our file should look like this finally.
/* src/app.js */import React from "react";
import logo from "./logo.svg";
import "./App.css";
import Sawo from "sawo";
function App() {
return (
<div
id="sawo-container"
style={{ height: "300px", width: "300px" }}
></div>
);
}export default App;
- Congratulations, but if you check your application on the browser, well we get a blank, nothing yet. Now it’s time, to call the SDK! :)
The Crux Point
We need to call the SDK when our div
with id as sawo-container
is rendered. So we are going to use theReact.useEffect()
hook in order to suffice this condition in the file src/app.js
.
/* src/app.js */import React from "react";
import logo from "./logo.svg";
import "./App.css";
import Sawo from "sawo";
function App() {
/*
This makes sure when the component is rendered,
it is called only once.
*/
React.useEffect(() => { }, []);
return (
<div
id="sawo-container"
style={{ height: "300px", width: "300px" }}
></div>
);
}export default App;
- It’s time to write the config for SAWO Authentication, as discussed in the 6th point in their docs here. We are going to put it inside the
useEffect
hook. Why? Because we want to execute this after the component is mounted on the screen. - After preparing the config, we are going to create a new SAWO instance with the
config
and we are going to execute theshowForm
method. Finally,useEffect
looks like this:
React.useEffect(() => {
var config = {
containerID: "sawo-container",
identifierType: "email",
apiKey: process.env.REACT_APP_API_SAWO,
onSuccess: (payload) => {
console.log(payload);
},
};
let sawo = new Sawo(config);
sawo.showForm();
}, []);
- Let’s head over to the application on the browser. Now you should notice the SAWO authentication asking for your email id. :)
Dashboard Connection.
- We are going to create a file
src/Dashboard.js
, which looks like this.
import React from "react";const Dashboard = (props) => { return (
<>
<h2>Private Dashboard</h2>
Email: {props.payload.identifier}
</>
);
};export default Dashboard;
Head over to src/app.js
and we are going to perform the following operations:
- We need to import our Dashboard Component in App.
- We need to create a state, which stores the payload after the user is authenticated.
Let’s do it.
/* src/app.js */import React from "react";
import logo from "./logo.svg";
import "./App.css";/* This imports Dashboard Component*/
import Dashboard from './dashboard'import Sawo from "sawo";
function App() {/* We are creating a state, using the useState hook. */let [payload, setPayload] = React.useState(false);/*
This makes sure when the component is rendered,
it is called only once.
*/
React.useEffect(() => {
var config = {
containerID: "sawo-container",
identifierType: "email",
apiKey: process.env.REACT_APP_API_SAWO,
onSuccess: (payload) => {
// console.log(payload);
setPayload(payload)
},
};
let sawo = new Sawo(config);
sawo.showForm();
}, []);
return (<>
<div
id="sawo-container"
style={{ height: "300px", width: "300px" }}
></div>
{payload && (
<>
<Dashboard payload={payload} />
</>
)}
</>
);
}export default App;
And that’s it. Hope you enjoyed reading this article.
More content at plainenglish.io